배달의 민족 앱에서 유저 등록 만큼 중요한 것이 바로 레스토랑 등록이다.
레스토랑은 가계주인이 등록 할 것이므로 일반 유저가 사용하는 부분이 아니다.
레스토랑은 유저관리 보다 필요한 정보가 많으므로 생각할게 좀더 많은 것 같다.
레스토랑 등록
Model package
PostRestaurantReq
package com.example.delivery.src.restaurant.model;
import lombok.*;
@Getter
@Setter
@AllArgsConstructor
@NoArgsConstructor(access = AccessLevel.PROTECTED)
public class PostRestaurantReq {
private String name;
private String delivery_category;
private String category;
private int minimumCost;
private int deliveryCost;
private int deliveryTime;
private String location;
private String operatingTime;
private String holiday;
private String explantion;
}
한 레스토랑 마다 등록하기 위해 필요한 정보이다.
PostRestaurantRes
package com.example.delivery.src.restaurant.model;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
@AllArgsConstructor
public class PostRestaurantRes {
private int restaurantIdx;
}
입력에대한 결과는 레스토랑 번호만 출력하도록 만들었다.
RestaurantController
/***
* 레스토랑 추가 API
* [POST] /restaurants
*/
@ResponseBody
@PostMapping("/regist")
public BaseResponse<PostRestaurantRes> registRestaurant(@RequestBody PostRestaurantReq postRestaurantReq){
//레스토랑 이름이 안들어오면 에러 발생
if(postRestaurantReq.getName() == null){
return new BaseResponse<>(BaseResponseStatus.POST_RESTAURANTS_EMPTY_NAME);
}
try{
PostRestaurantRes postRestaurantRes = restaurantService.registRestaurant(postRestaurantReq);
return new BaseResponse<>(postRestaurantRes);
} catch(BaseException exception){
return new BaseResponse<>(exception.getStatus());
}
}
유저를 추가하는 부분과 매우 비슷하다.레스토랑 이름이 안들어오면 에러를 발생하게 만들었다.
RestaurantService
public PostRestaurantRes registRestaurant (PostRestaurantReq postRestaurantReq) throws BaseException {
try{
int restaurantIdx = restaurantDao.registRestaurant(postRestaurantReq);
return new PostRestaurantRes(restaurantIdx);
} catch (Exception exception){
throw new BaseException(BaseResponseStatus.DATABASE_ERROR);
}
}
```
RestaurantDao
public PostRestaurantRes registRestaurant (PostRestaurantReq postRestaurantReq) throws BaseException {
try{
int restaurantIdx = restaurantDao.registRestaurant(postRestaurantReq);
return new PostRestaurantRes(restaurantIdx);
} catch (Exception exception){
throw new BaseException(BaseResponseStatus.DATABASE_ERROR);
}
}
실행결과
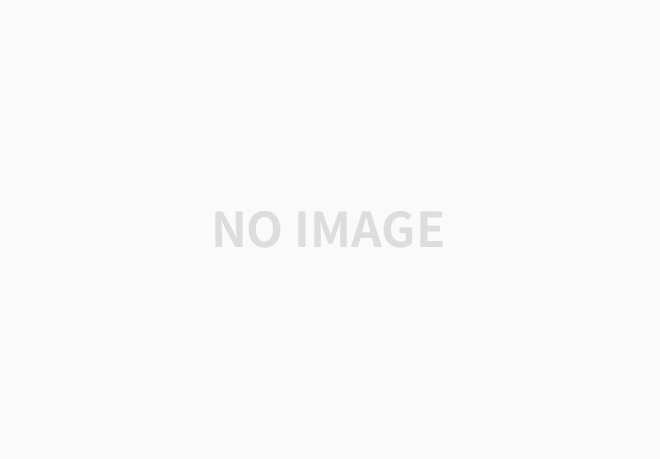
간단하게 가계를 등록하는 API를 구현해보았다.
'혼자하는 프로젝트 > 배달의 민족 클론코딩' 카테고리의 다른 글
레스토랑 관리 - 레스토랑 조회(Paging 처리) (0) | 2022.12.19 |
---|---|
유저관리 - 회원정보 변경 및 조회 (0) | 2022.12.19 |
유저관리 - 로그인 (0) | 2022.12.16 |
유저 관리 - 회원 가입 (0) | 2022.12.14 |
유저 관리 - 준비 단계 (0) | 2022.12.14 |